Matlab’s built-in editor, like most other Matlab GUI, is Java-based. As such, it can easily be accessed programmatically. ImageAnalyst, a well-respected member of the Matlab community and a frequent CSSM (newsgroup) and FEX (File Exchange) contributor, recently asked whether it is possible to retrieve the name of the Editor’s currently edited file. The answer is that this is very easy, but I decided to use this opportunity to show how other interesting things can be done with the Editor.
Before we start, it should be made clear that this entire article relies on MathWorks internal implementation of the Editor and Desktop, which may change without prior notice in future Matlab releases. The code below appears to work under Matlab 6 & 7, but users who rely on forward compatibility should be aware of this warning.
We start by retrieving the Editor handle. This can be done in a number of ways. The easiest is via the Matlab desktop:
try % Matlab 7 desktop = com.mathworks.mde.desk.MLDesktop.getInstance; jEditor = desktop.getGroupContainer('Editor').getTopLevelAncestor; % we get a com.mathworks.mde.desk.MLMultipleClientFrame object catch % Matlab 6 % Unfortunately, we can't get the Editor handle from the Desktop handle in Matlab 6: %desktop = com.mathworks.ide.desktop.MLDesktop.getMLDesktop; % So here's the workaround for Matlab 6: openDocs = com.mathworks.ide.editor.EditorApplication.getOpenDocuments; % a java.util.Vector firstDoc = openDocs.elementAt(0); % a com.mathworks.ide.editor.EditorViewContainer object jEditor = firstDoc.getParent.getParent.getParent; % we get a com.mathworks.mwt.MWTabPanel or com.mathworks.ide.desktop.DTContainer object end
Now that we have the Editor handle, let’s retrieve its currently open (active) file name from the Editor’s title:
title = jEditor.getTitle; currentFilename = char(title.replaceFirst('Editor - ',''));
The entire list of open file names can be retrieved in several ways:
% Alternative #1: edhandle = com.mathworks.mlservices.MLEditorServices; allEditorFilenames = char(edhandle.builtinGetOpenDocumentNames); % Alternative #2: openFiles = desktop.getWindowRegistry.getClosers.toArray.cell; allEditorFilenames = cellfun(@(c)c.getTitle.char,openFiles,'un',0);
At the top-level Editor-window level, we can prevent its resizing, update its status bar, modify its toolbar/menu-bar, control docking and do other similar fun things:
% Actions via built-in methods: jEditor.setResizable(0); jEditor.setStatusText('testing 123...'); jEditor.setTitle('This is the Matlab Editor'); % Equivalent actions via properties: set(jEditor, 'Resizable', 'off'); set(jEditor, 'StatusText', 'testing 123...'); set(jEditor, 'Title', 'This is the Matlab Editor');
Actually, the jEditor handle has over 300 invokable methods and close to 200 properties that we can get/set. Perhaps the easiest way to find interesting things we can programmatically do with the Editor handle, is to use my UIInspect utility on the File Exchange:
uiinspect(jEditor); % or: jEditor.uiinspect
Clik here to view.
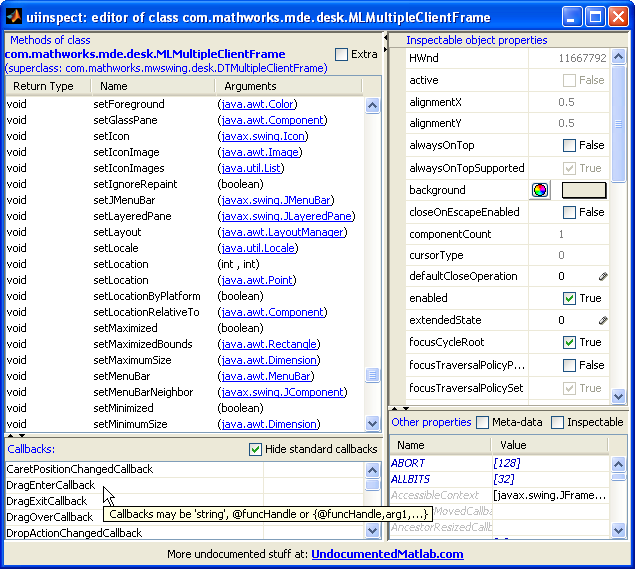
Matlab Editor methods, callbacks and properties as seen by uiinspect
(click to zoom)
The Editor handle is actually a container for many internal panels (toolbars etc.) and documents. The entire object hierarchy can be seen with another of my File Exchange utilities, FindJObj:
findjobj(jEditor); % or: jEditor.findjobj
Clik here to view.
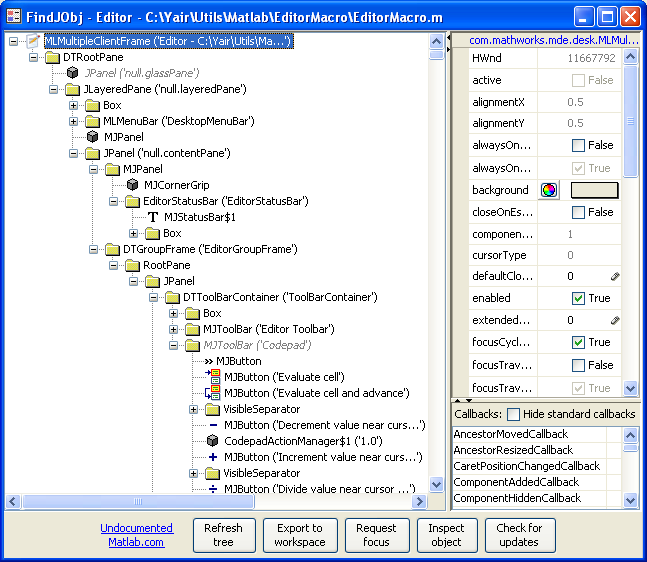
Matlab Editor object hierarchy as seen by findjboj (click to zoom)
We can modify text within the open Editor documents, and instrument these document to handle event callbacks. To see how, I refer users to my EditorMacro utility on the Matlab File Exchange.
If you find some other nifty and/or useful things that can be done using the Editor handle, please post them in the comments section below.
Related posts:
- EditorMacro – assign a keyboard macro in the Matlab editor EditorMacro is a new utility that enables setting keyboard macros in the Matlab editor. this post details its inner workings....
- Non-textual editor actions The UIINSPECT utility can be used to expand EditorMacro capabilities to non-text-insertion actions. This is how:...
- Variables Editor scrolling The Matlab Variables Editor can be accessed to provide immediate scrolling to a specified cell location. ...
- Recovering previous editor state Recovering the previous state of the Matlab editor and its loaded documents is possible using a built-in backup config file. ...